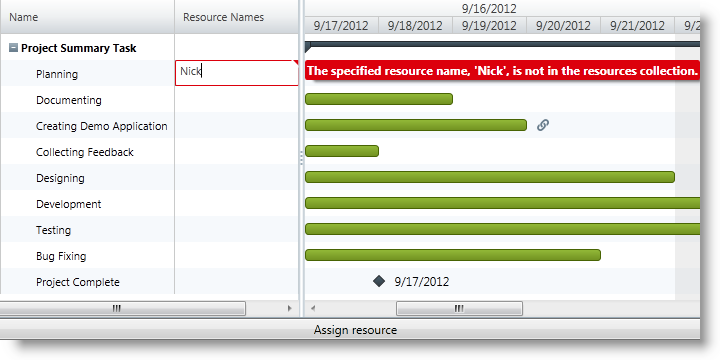
This topic explains how you create resources available for a project and assign them to the project’s tasks using the xamGantt™ control.
The following topics are prerequisites to understanding this topic:
This topic contains the following sections:
The following table lists the configurable aspects relating to the resources in the xamGantt control. Additional details follow later in the topic.
You can create resources and add them to a project’s resource collection in the xamGantt control. After that, you can assign resources to the tasks from the available project’s resources or create new ones via the user interface.
The following table maps the example configuration to property settings.
The example code below demonstrates how to create a resource, add it the project’s available resources collection and disabling the addition of new resources via the xamGantt UI.
The screenshot illustrates the error tooltip that appears when a user attempts to assign a new unrecognized resource to a task:
In C#:
ProjectSettings settings = new ProjectSettings();
// Disable adding resources through the xamGantt UI
// and via the ProjectTask ResourcesText property
settings.AutoAddNewResources = false;
this.gantt.Project.Settings = settings;
// Create ProjectResource with display name and unique ID
ProjectResource projectResource = new ProjectResource();
projectResource.DisplayName = "John Smith";
projectResource.UniqueId = "dev-jsmith-45673";
// Add created resource to the project ResourceItems collection
this.gantt.Project.ResourceItems.Add(projectResource);
In Visual Basic:
Dim settings As New ProjectSettings()
' Disable adding resources through the xamGantt UI and via the ProjectTask ResourcesText property
settings.AutoAddNewResources = False
Me.gantt.Project.Settings = settings
' Create ProjectResource with display name and unique ID
Dim projectResource As New ProjectResource()
projectResource.DisplayName = "John Smith"
projectResource.UniqueId = "dev-jsmith-45673"
' Add created resource to the project ResourceItems collection
Me.gantt.Project.ResourceItems.Add(projectResource)
You can create resources and assign them to tasks using the xamGantt control. You may add as many resources to a task as required, but you may not add the same resource more than once to the same tasks.
The following table maps the desired configuration to property settings.
The example code demonstrates how to assign a resource to the currently active task on a Button Click event. Additionally, it depicts the error dialog box resulting from attempting to assign a resource to a task more than once:
In C#:
private void Btn_AddResource_Click(object sender, RoutedEventArgs e)
{
// Create a ProjectTaskResource for the available project resource with specified unique ID
ProjectTaskResource taskResource = new ProjectTaskResource("dev-jsmith-45673");
try
{
// Assign the resource to the task
this.gantt.ActiveRow.Value.Task.Resources.Add(taskResource);
}
catch (Exception exc)
{
// Show a message if an exception occurs
MessageBox.Show(exc.Message);
}
}
In Visual Basic:
Private Sub Btn_AddResource_Click(sender As Object, e As RoutedEventArgs)
' Create a ProjectTaskResource for the available project resource with specified unique ID
Dim taskResource As New ProjectTaskResource("dev-jsmith-45673")
Try
' Assign the resource to the task
Me.gantt.ActiveRow.Value.Task.Resources.Add(taskResource)
Catch exc As Exception
' Show a message if an exception occurs
MessageBox.Show(exc.Message)
End Try
End Sub
This example demonstrates error handling in the event that a user attempts to enter an unrecognized resource that is not included as part of the project’s available resources.
Use the Project MissingResourceWarning event and handle it to process this error.
The example below demonstrates how to handle the addition of an unrecognized resource error event:
In C#:
…
this.gantt.Project.MissingResourceWarning +=
new EventHandler<MissingResourceWarningEventArgs>(Project_MissingResourceWarning);
…
In C#:
private void Project_MissingResourceWarning(object sender, MissingResourceWarningEventArgs e)
{
// Show a message if an exception occurs
MessageBox.Show(string.Format("The {0} resource is an unrecognized resource.", e.ResourceName));
}
In Visual Basic:
…
Me.gantt.Project.MissingResourceWarning = New EventHandler(Of MissingResourceWarningEventArgs)(Project_MissingResourceWarning)
…
In Visual Basic:
Private Sub Project_MissingResourceWarning(sender As Object, e As MissingResourceWarningEventArgs)
' Show a message if an exception occurs
MessageBox.Show(String.Format("The {0} resource is an unrecognized resource.", e.ResourceName))
End Sub
The following topics provide additional information related to this topic.