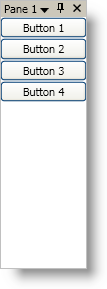
The ContentPane object derives from HeaderedContentControl; therefore, using it will be similar to any headered content control found in Microsoft® Windows® Presentation Foundation. Just like any content control in Windows Presentation Foundation, you can set the Content property of a content pane to an instance of an object. In many cases, you will use a layout container, such as a StackPanel, as the root element — adding additional elements to the layout container.
Not only can you set the Content property of a content pane, but you can also set the Header property to display text in the pane’s header. If you unpin and hide a content pane, the tab header will automatically display the header text. You can override this default behavior by setting the content pane’s TabHeader property.
You will also find the following properties that restrict docking related behaviors of the content pane:
The following example code demonstrates how to add content to a content pane.
In XAML:
... <igDock:XamDockManager Name="xamDockManager1"> <igDock:XamDockManager.Panes> <igDock:SplitPane> <igDock:ContentPane Header="Pane 1"> <StackPanel> <Button Content="Button 1" /> <Button Content="Button 2" /> <Button Content="Button 3" /> <Button Content="Button 4" /> </StackPanel> </igDock:ContentPane> </igDock:SplitPane> </igDock:XamDockManager.Panes> </igDock:XamDockManager> ...
In Visual Basic:
Imports Infragistics.Windows.DockManager ... 'Create a SplitPane object Dim splitPane1 As New SplitPane() 'Add the SplitPane to xamDockManager's Panes collection Me.xamDockManager1.Panes.Add(splitPane1) 'Create a ContentPane and set its Header property Dim buttonPane As New ContentPane() buttonPane.Header = "Pane 1" 'Add the ContentPane to the SplitPane's Panes collection splitPane1.Panes.Add(buttonPane) 'Create a StackPanel Dim panel As New StackPanel() 'Set the ContentPane's Content property to the panel buttonPane.Content = panel 'Create four Button controls and add it to the StackPanel's Children collection For i As Integer = 1 To 4 Dim b As New Button() b.Content = "Button " + i.ToString() panel.Children.Add(b) Next ...
In C#:
using Infragistics.Windows.DockManager; ... //Create a SplitPane object SplitPane splitPane1 = new SplitPane(); //Add the SplitPane to xamDockManager's Panes collection this.xamDockManager1.Panes.Add(splitPane1); //Create a ContentPane and set its Header property ContentPane buttonPane = new ContentPane(); buttonPane.Header = "Pane 1"; //Add the ContentPane to the SplitPane's Panes collection splitPane1.Panes.Add(buttonPane); //Create a StackPanel StackPanel panel = new StackPanel(); //Set the ContentPane's Content property to the panel buttonPane.Content = panel; //Create four Button controls and add it to the StackPanel's Children collection for (int i = 1; i < 5; i++) { Button b = new Button(); b.Content = "Button " + i.ToString(); panel.Children.Add(b); } ....